Table of Contents
How do you count NaN in Python?
You can use the following syntax to count NaN values in Pandas DataFrame:
- (1) Count NaN values under a single DataFrame column: df[‘column name’].isna().sum()
- (2) Count NaN values under an entire DataFrame: df.isna().sum().sum()
- (3) Count NaN values across a single DataFrame row: df.loc[[index value]].isna().sum().sum()
Does pandas count () count NaN?
How to count the NaN values in a column in a Python Pandas DataFrame? To count the NaN values in a column in a Pandas DataFrame, we can use the isna() method with sum.
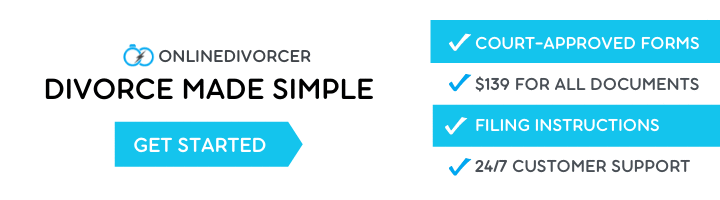
Does count include NaN?
The count property directly gives the count of non-NaN values in each column. So, we can get the count of NaN values, if we know the total number of observations. The isnull() function returns a dataset containing True and False values.
Which attribute is used with series to count the total number of NaN values in Python?
isna(). sum() to count the total number of NaN values in the column col of the DataFrame .
What is NaN Python?
NaN , standing for not a number, is a numeric data type used to represent any value that is undefined or unpresentable. For example, 0/0 is undefined as a real number and is, therefore, represented by NaN.

How do you find the NaN of a data frame?
Here are 4 ways to check for NaN in Pandas DataFrame:
- (1) Check for NaN under a single DataFrame column: df[‘your column name’].isnull().values.any()
- (2) Count the NaN under a single DataFrame column: df[‘your column name’].isnull().sum()
- (3) Check for NaN under an entire DataFrame: df.isnull().values.any()
How do you find the NaN of a DataFrame?
What does count () do in pandas?
Pandas DataFrame count() Method The count() method counts the number of not empty values for each row, or column if you specify the axis parameter as axis=’columns’ , and returns a Series object with the result for each row (or column).
Which can be used to count total NaN values in a Dataframe?
isna() produces Boolean Series where the number of True is the number of NaN , and df. isna(). sum() adds False and True replacing them respectively by 0 and 1. Therefore this indirectly counts the NaN , where a simple count would just return the length of the column.
Why is NaN a number?
NaN stands for Not a Number. It is a value of numeric data types (usually floating point types, but not always) that represents the result of an invalid operation such as dividing by zero. Although its names says that it’s not a number, the data type used to hold it is a numeric type.
How do I find NaN values?
The math. isnan() method checks whether a value is NaN (Not a Number), or not. This method returns True if the specified value is a NaN, otherwise it returns False.
What is NaN value?
What are NaN values? NaN or Not a Number are special values in DataFrame and numpy arrays that represent the missing of value in a cell. In programming languages they are also represented, for example in Python they are represented as None value.
Where is NaN Python?
In Python, the float type has nan (not a number). nan is defined by the IEEE 754 floating-point standard.
How do you count data in Python?
Summary:
- The count() is a built-in function in Python. It will return you the count of a given element in a list or a string.
- In the case of a list, the element to be counted needs to be given to the count() function, and it will return the count of the element.
- The count() method returns an integer value.
How do I count a column in Python?
You can try different methods to get the number of rows and columns of the dataframe:
- len(df)
- len(df. index)
- df. shape[0]
- df[df. columns[0]]. count()
- df. count()
- df. size.
What is NaN function?
The isNaN() method returns true if a value is NaN. The isNaN() method converts the value to a number before testing it.
What is NaN in Python pandas?
The official documentation for pandas defines what most developers would know as null values as missing or missing data in pandas. Within pandas, a missing value is denoted by NaN .
How do you count values in a column?
Count Numbers, All Data, or Blank Cells
- Enter the sample data on your worksheet.
- In cell A7, enter an COUNT formula, to count the numbers in column A: =COUNT(A1:A5)
- Press the Enter key, to complete the formula.
- The result will be 3, the number of cells that contain numbers.
What is a NaN value?
NaN stands for Not A Number and is one of the common ways to represent the missing value in the data. It is a special floating-point value and cannot be converted to any other type than float. NaN value is one of the major problems in Data Analysis.
How do you check for NaN values in Python?
The most common method to check for NaN values is to check if the variable is equal to itself. If it is not, then it must be NaN value. Another property of NaN which can be used to check for NaN is the range.
How do you count Nan strings?
If they’re real floating-point NaNs, ordinary “count this thing” answers will be foiled by NaN != NaN. – user2357112 supports Monica Mar 24 ’17 at 17:21 It turns out they are ‘NaN’ strings and the list.count(‘NaN’) method works.
How to get the Count of Nan in a pandas Dataframe?
To get the count of NaN in a pandas dataframe, the simplest way is to use the pandas isnull () function and pandas sum () function. When working with data as a data science or data analyst, it’s important to be able to find the basic descriptive statistics of a set of data.
What is Nanan in Python?
NaN stands for Not A Number and is one of the common ways to represent the missing value in the data. It is a special floating-point value and cannot be converted to any other type than float.